Spring 프로젝트에 Mybatis 설정
1. src/main/resources에 mappers 폴더 생성 후 timeMapper.xml 파일 생성
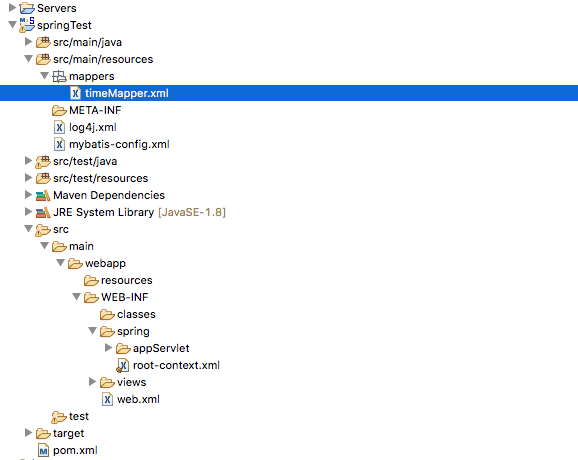
2. timeMapper.xml에 DTD를 추가 후 SQL문 작성
<?xml version="1.0" encoding="UTF-8"?>
3. root-context.xml의 sqlSessionFactory bean에 아래의 코드 추가
4. src/main/java 폴더에 dao 패키지를 생성하고 TimeDAO 인터페이스 생성
5. TimeDAO의 구현 클래스로 TimeDAOImpl 클래스 생성
6. persistence 패키지를 Spring에 bean으로 등록
7. src/test/java 폴더에 있는 패키지에 TimeDAOTester 클래스 생성
8. 웹 화면 호출
#서버를 구동시키고 http://localhost:8080/getTime 에 접속을 하면 현재 시간이 출력된다.
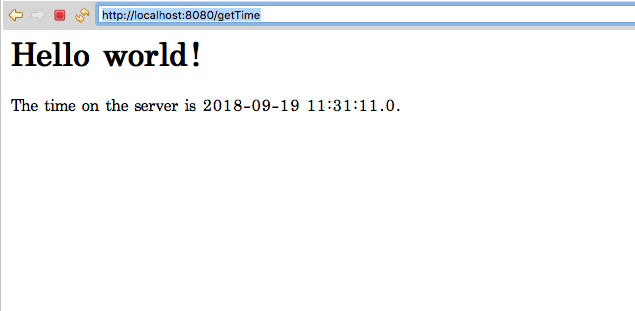
1. src/main/resources에 mappers 폴더 생성 후 timeMapper.xml 파일 생성
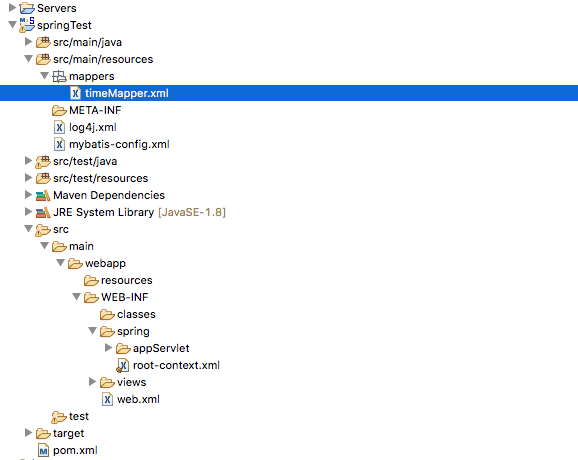
2. timeMapper.xml에 DTD를 추가 후 SQL문 작성
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="TimeMapper">
<select id="getTime" resultType="String">
select sysdate()
</select>
</mapper>
<bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean">
<property name="dataSource" ref="dataSource" />
<property name="configLocation" value="classpath:/mybatis-config.xml"></property>
<property name="mapperLocations" value="classpath:mappers/**/*Mapper.xml"></property>
</bean>
4. src/main/java 폴더에 dao 패키지를 생성하고 TimeDAO 인터페이스 생성
public interface TimeDao {
public String getTime();
}
5. TimeDAO의 구현 클래스로 TimeDAOImpl 클래스 생성
@Repository
public class TimeDAOImpl implements TimeDao {
@Inject
private SqlSession sqlSession;
private static final String NAMESPACE = "TimeMapper.";
@Override
public String getTime() {
return sqlSession.selectOne(NAMESPACE + "getTime");
}
}
6. persistence 패키지를 Spring에 bean으로 등록
<context:component-scan base-package="com.fogs.myapp.dao" />
7. src/test/java 폴더에 있는 패키지에 TimeDAOTester 클래스 생성
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(locations = { "file:src/main/webapp/WEB-INF/spring/root-context.xml" })
public class TimeDAOTester {
@Inject
private TimeDao dao;
@Test
public void testTime() throws Exception{
System.out.println(dao.getTime());
}
}
8. 웹 화면 호출
@Inject
private TimeDao dao;
@RequestMapping(value = "/getTime", method = RequestMethod.GET)
public String getTime(Locale locale, Model model) {
logger.info("Welcome home!");
model.addAttribute("serverTime", dao.getTime());
return "home";
}
#서버를 구동시키고 http://localhost:8080/getTime 에 접속을 하면 현재 시간이 출력된다.
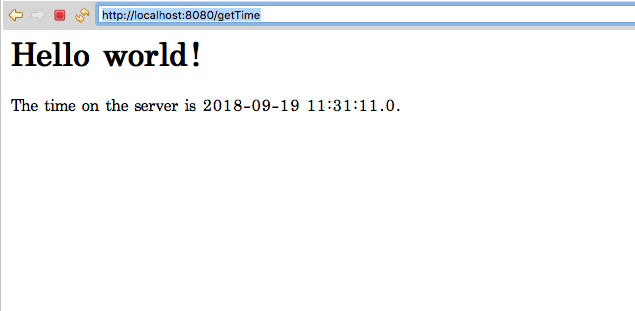
댓글
댓글 쓰기